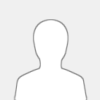 |
// Example to read channel 1 and output to MQTT server
// Patched together from the other examples
// Install EmonLib and PubSubClient
#include <ETH.h>
#include <PubSubClient.h>
#include "Arduino.h"
#include "EmonLib.h"
EnergyMonitor emon1;
#define ETH_ADDR 0
#define ETH_POWER_PIN -1
#define ETH_MDC_PIN 23
#define ETH_MDIO_PIN 18
#define ETH_TYPE ETH_PHY_LAN8720
#define ETH_CLK_MODE ETH_CLOCK_GPIO17_OUT
// WiFiUDP Udp; //Create UDP object
unsigned int localUdpPort = 4196; //local port
IPAddress local_ip(10, 0, 0, 143);
IPAddress gateway(10, 0, 0, 1);
IPAddress subnet(255, 255, 255, 0);
IPAddress dns(10, 0, 0, 1);
#define s0 32
#define s1 33
#define s2 13
#define s3 16
#define IN3 35
int toggleState_1 = 1; //Define integer to remember the toggle state for relay 1
int toggleState_2 = 1; //Define integer to remember the toggle state for relay 2
int toggleState_3 = 1; //Define integer to remember the toggle state for relay 3
int toggleState_4 = 1; //Define integer to remember the toggle state for relay 4
// Update these with values suitable for your network.
const char* mqttServer = "10.0.0.16";
const char* mqttUserName = ""; // MQTT username
const char* mqttPwd = ""; // MQTT password
const char* clientID = "clientId"; // client id
WiFiClient espClient;
PubSubClient client(espClient);
int value = 0;
void setup_ethernet() {
ETH.begin(ETH_ADDR, ETH_POWER_PIN, ETH_MDC_PIN, ETH_MDIO_PIN, ETH_TYPE, ETH_CLK_MODE); //start with ETH
if (ETH.config(local_ip, gateway, subnet, dns, dns) == false) {
Serial.println("LAN8720 Configuration failed.");
}else{Serial.println("LAN8720 Configuration success.");}
Serial.println("Connected");
Serial.print("IP Address:");
Serial.println(ETH.localIP());
}
void reconnect() {
while (!client.connected()) {
if (client.connect(clientID, mqttUserName, mqttPwd)) {
Serial.println("MQTT connected");
// ... and resubscribe
}
else
{
Serial.print("failed, rc=");
Serial.print(client.state());
Serial.println(" try again in 5 seconds");
// Wait 5 seconds before retrying
delay(5000);
}
}
}
void callback(char* topic, byte* payload, unsigned int length) {
Serial.print("Message arrived [");
Serial.print(topic);
Serial.print("] ");
Serial.println("");
}
String byteToHexString(uint8_t byte) {
String hexStr = String(byte, HEX);
if (hexStr.length() == 1) {
hexStr = "0" + hexStr;
}
return hexStr;
}
void setup() {
Serial.begin(115200);
pinMode(s0,OUTPUT);
pinMode(s1,OUTPUT);
pinMode(s2,OUTPUT);
pinMode(s3,OUTPUT);
pinMode(IN3,INPUT);
setup_ethernet();
client.setBufferSize(2048);
client.setServer(mqttServer, 1883);
client.setCallback(callback);
emon1.current(IN3, 20); // ADC_PIN is the pin where SCT013 is connected, 20 amps
}
void loop() {
if (!client.connected()) {
reconnect();
}
client.loop();
Serial.println("done loop");
// Set Low/High to flag for the input to read from: 0000 = chanel 1 to 1111 chanel 16
digitalWrite(s0,LOW);
digitalWrite(s1,LOW);
digitalWrite(s2,LOW);
digitalWrite(s3,LOW);
if(analogRead(IN3)!=0){
double Irms = emon1.calcIrms(1480); // Calculate Irms only, 1480 is num samples per second
Irms = Irms * 110; // 110v
Serial.printf("A3 on CH1=%d\n",Irms);
String val = "val 1 " + String(Irms);
client.publish("sensorData", val.c_str());
}
delay(2000);
}
|